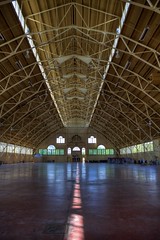
© 2011 Darren DeRidder.
// hello.js var greeting="Hello World!"; var farewell="Goodbye Cruel World!"; var greet = function(){ return greeting; }; var leave = function() { return farewell; }; exports.greet = greet; exports.leave = leave;
// app.js var hello=require('./lib/hello.js'); console.log(hello.greet()); console.log(hello.leave());
npm install -g expresso
mkdir test vi test/hello.test.js
// test/hello.test.js var assert = require('assert'), hello = require('hello.js); module.exports = { 'greet()': function() { assert.equal('Hello World!', hello.greet()); } };
$ expresso -I lib %100 1 testsWonderful. Only, we didn't test the part where we say goodbye. Expresso has built-in code coverage reporting that we can enable with the "-c" flag:
$ expresso -I lib -c Test Coverage +------------------------------------------+----------+------+------+--------+ | filename | coverage | LOC | SLOC | missed | +------------------------------------------+----------+------+------+--------+ | hello.js | 87.50 | 10 | 8 | 1 | +------------------------------------------+----------+------+------+--------+ | 87.50 | 10 | 8 | 1 | +----------+------+------+--------+ hello.js: 1 | 1 | var greeting = "Hello World!"; 2 | 1 | var farewell = "Goodbye Cruel World!"; 3 | 1 | var greet = function() { 4 | 1 | return greeting; 5 | | }; 6 | 1 | var leave = function() { 7 | 0 | return farewell; 8 | | }; 9 | 1 | exports.greet = greet; 10 | 1 | exports.leave = leave;
// test/hello.test.js var assert = require('assert'), hello = require('hello.js'); module.exports = { 'greet()': function() { assert.equal('Hello World!', hello.greet()); }, 'leave()': function() { assert.equal('Goodbye Cruel World!', hello.leave()); } };
$ expresso -I lib -c Test Coverage +------------------------------------------+----------+------+------+--------+ | filename | coverage | LOC | SLOC | missed | +------------------------------------------+----------+------+------+--------+ | hello.js | 100.00 | 10 | 8 | 0 | +------------------------------------------+----------+------+------+--------+ | 100.00 | 10 | 8 | 0 | +----------+------+------+--------+ hello.js: 1 | 1 | var greeting = "Hello World!"; 2 | 1 | var farewell = "Goodbye Cruel World!"; 3 | 1 | var greet = function() { 4 | 1 | return greeting; 5 | | }; 6 | 1 | var leave = function() { 7 | 1 | return farewell; 8 | | }; 9 | 1 | exports.greet = greet; 10 | 1 | exports.leave = leave;
$ node > console.log("Hello World!"); Hello World!
// hello.js console.log("Hello World!")
$ node hello.js Hello World!
// app.js require('./hello.js');
// hello.js console.log("Hello World!");
$ node app.js Hello World!
#!/usr/bin/env node // app.js require('./hello.js');
chmod u+x app.js
$ ./app.js Hello World!
// hello.js var greeting = "Hello World!"; var greet = function() { console.log(greeting); } exports.greet = greet;
#!/usr/bin/env node // app.js var mygreet = require('./hello.js').greet; mygreet();
I told a friend of mine that I wasn't really happy with the amount of time that gets taken up by Slack and "communication and sched...